Interview :: C++
C++ is an object-oriented programming language created by Bjarne Stroustrup. It was released in 1985.
C++ is a superset of C with the major addition of classes in C language.
Initially, Stroustrup called the new language "C with classes". However, after sometime the name was changed to C++. The idea of C++ comes from the C increment operator ++.
C++ doesn't only maintains all aspects from C language, it also simplifies memory management and adds several features like:
- C++ is a highly portable language means that the software developed using C++ language can run on any platform.
- C++ is an object-oriented programming language which includes the concepts such as classes, objects, inheritance, polymorphism, abstraction.
- C++ has the concept of inheritance. Through inheritance, one can eliminate the redundant code and can reuse the existing classes.
- Data hiding helps the programmer to build secure programs so that the program cannot be attacked by the invaders.
- Message passing is a technique used for communication between the objects.
- C++ contains a rich function library.
What is the difference between C and C++?
Following are the differences between C and C++:
C | C++ |
---|---|
C language was developed by Dennis Ritchie. | C++ language was developed by Bjarne Stroustrup. |
C is a structured programming language. | C++ supports both structural and object-oriented programming language. |
C is a subset of C++. | C++ is a superset of C. |
In C language, data and functions are the free entities. | In the C++ language, both data and functions are encapsulated together in the form of a project. |
C does not support the data hiding. Therefore, the data can be used by the outside world. | C++ supports data hiding. Therefore, the data cannot be accessed by the outside world. |
C supports neither function nor operator overloading. | C++ supports both function and operator overloading. |
In C, the function cannot be implemented inside the structures. | In the C++, the function can be implemented inside the structures. |
Reference variables are not supported in C language. | C++ supports the reference variables. |
C language does not support the virtual and friend functions. | C++ supports both virtual and friend functions. |
In C, scanf() and printf() are mainly used for input/output. | C++ mainly uses stream cin and cout to perform input and output operations. |
The class is a user-defined data type. The class is declared with the keyword class. The class contains the data members, and member functions whose access is defined by the three modifiers are private, public and protected. The class defines the type definition of the category of things. It defines a datatype, but it does not define the data it just specifies the structure of data.
You can create N number of objects from a class.
The various OOPS concepts in C++ are:
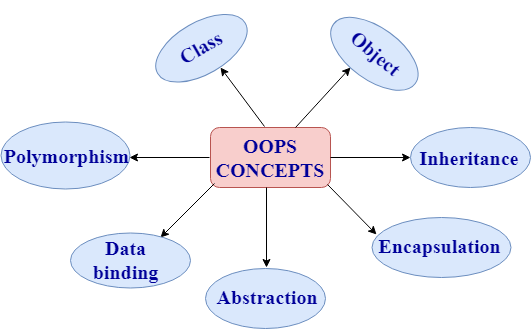
- Class:
The class is a user-defined data type which defines its properties and its functions. For example, Human being is a class. The body parts of a human being are its properties, and the actions performed by the body parts are known as functions. The class does not occupy any memory space. Therefore, we can say that the class is the only logical representation of the data.
The syntax of declaring the class:
- Object:
An object is a run-time entity. An object is the instance of the class. An object can represent a person, place or any other item. An object can operate on both data members and member functions. The class does not occupy any memory space. When an object is created using a new keyword, then space is allocated for the variable in a heap, and the starting address is stored in the stack memory. When an object is created without a new keyword, then space is not allocated in the heap memory, and the object contains the null value in the stack.
The syntax for declaring the object:
- Inheritance:
Inheritance provides reusability. Reusability means that one can use the functionalities of the existing class. It eliminates the redundancy of code. Inheritance is a technique of deriving a new class from the old class. The old class is known as the base class, and the new class is known as derived class.
Syntax
Note: The visibility-mode can be public, private, protected.
- Encapsulation:
Encapsulation is a technique of wrapping the data members and member functions in a single unit. It binds the data within a class, and no outside method can access the data. If the data member is private, then the member function can only access the data.
- Abstraction:
Abstraction is a technique of showing only essential details without representing the implementation details. If the members are defined with a public keyword, then the members are accessible outside also. If the members are defined with a private keyword, then the members are not accessible by the outside methods.
- Data binding:
Data binding is a process of binding the application UI and business logic. Any change made in the business logic will reflect directly to the application UI.
- Polymorphism:
Polymorphism means multiple forms. Polymorphism means having more than one function with the same name but with different functionalities. Polymorphism is of two types:
- Static polymorphism is also known as early binding.
- Dynamic polymorphism is also known as late binding.
Polymorphism: Polymorphism means multiple forms. It means having more than one function with the same function name but with different functionalities.
Polymorphism is of two types:
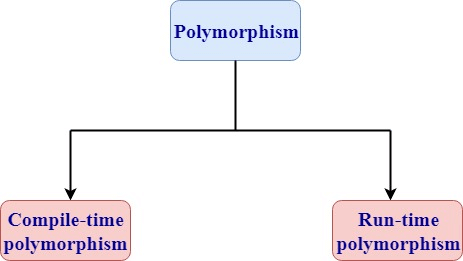
- Runtime polymorphism
Runtime polymorphism is also known as dynamic polymorphism. Function overriding is an example of runtime polymorphism. Function overriding means when the child class contains the method which is already present in the parent class. Hence, the child class overrides the method of the parent class. In case of function overriding, parent and child class both contains the same function with the different definition. The call to the function is determined at runtime is known as runtime polymorphism.
Let's understand this through an example:
Output:
javaTpoint tutorial
- Compile time polymorphism
Compile-time polymorphism is also known as static polymorphism. The polymorphism which is implemented at the compile time is known as compile-time polymorphism. Method overloading is an example of compile-time polymorphism.
Method overloading: Method overloading is a technique which allows you to have more than one function with the same function name but with different functionality.
Method overloading can be possible on the following basis:
- The return type of the overloaded function.
- The type of the parameters passed to the function.
- The number of parameters passed to the function.
Let's understand this through an example:
Output:
6 24
- In the above example, mul() is an overloaded function with the different number of parameters.
- The namespace is a logical division of the code which is designed to stop the naming conflict.
- The namespace defines the scope where the identifiers such as variables, class, functions are declared.
- The main purpose of using namespace in C++ is to remove the ambiguity. Ambiquity occurs when the different task occurs with the same name.
- For example: if there are two functions exist with the same name such as add(). In order to prevent this ambiguity, the namespace is used. Functions are declared in different namespaces.
- C++ consists of a standard namespace, i.e., std which contains inbuilt classes and functions. So, by using the statement "using namespace std;" includes the namespace "std" in our program.
- Syntax of namespace:
Syntax of accessing the namespace variable:
Let's understand this through an example:
Output:
10
A token in C++ can be a keyword, identifier, literal, constant and symbol.
Bjarne Stroustrup.
Following are the operations that can be performed on pointers:
- Incrementing or decrementing a pointer: Incrementing a pointer means that we can increment the pointer by the size of a data type to which it points.
There are two types of increment pointers:
1. Pre-increment pointer: The pre-increment operator increments the operand by 1, and the value of the expression becomes the resulting value of the incremented. Suppose ptr is a pointer then pre-increment pointer is represented as ++ptr.
Let's understand this through an example:
Output:
Value of *ptr is : 1 Value of *++ptr : 2
2. Post-increment pointer: The post-increment operator increments the operand by 1, but the value of the expression will be the value of the operand prior to the incremented value of the operand. Suppose ptr is a pointer then post-increment pointer is represented as ptr++.
Let's understand this through an example:
Output:
Value of *ptr is : 1 Value of *ptr++ : 1
- Subtracting a pointer from another pointer: When two pointers pointing to the members of an array are subtracted, then the number of elements present between the two members are returned.