Interview :: python
Python was created by Guido van Rossum, and released in 1991.
It is a general-purpose computer programming language. It is a high-level, object-oriented language which can run equally on different platforms such as Windows, Linux, UNIX, and Macintosh. It is widely used in data science, machine learning and artificial intelligence domain.
It is easy to learn and require less code to develop the applications.
It is widely used for:
- Web development (server-side).
- Software development.
- Mathematics.
- System scripting.
Why Python?
- Python is compatible with different platforms like Windows, Mac, Linux, Raspberry Pi, etc.
- Python has a simple syntax as compared to other languages.
- Python allows a developer to write programs with fewer lines than some other programming languages.
- Python runs on an interpreter system, means that the code can be executed as soon as it is written. It helps to provide a prototype very quickly.
- Python can be described as a procedural way, an object-orientated way or a functional way.
Python is used in various software domains some application areas are given below.
- Web and Internet Development
- Games
- Scientific and computational applications
- Language development
- Image processing and graphic design applications
- Enterprise and business applications development
- Operating systems
- GUI based desktop applications
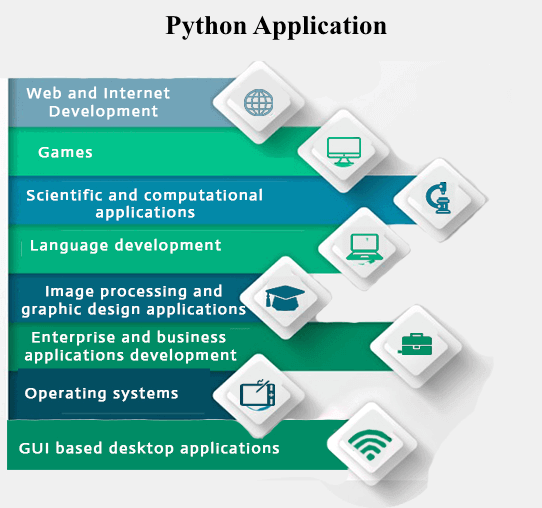
Python provides various web frameworks to develop web applications. The popular python web frameworks are Django, Pyramid, Flask.
Python's standard library supports for E-mail processing, FTP, IMAP, and other Internet protocols.
Python's SciPy and NumPy helps in scientific and computational application development.
Python's Tkinter library supports to create a desktop based GUI applications.
- Interpreted
- Free and open source
- Extensible
- Object-oriented
- Built-in data structure
- Readability
- High-Level Language
- Cross-platform
Interpreted: Python is an interpreted language. It does not require prior compilation of code and executes instructions directly. - Free and open source: It is an open source project which is publicly available to reuse. It can be downloaded free of cost.
- Portable: Python programs can run on cross platforms without affecting its performance.
- Extensible: It is very flexible and extensible with any module.
- Object-oriented: Python allows to implement the Object Oriented concepts to build application solution.
- Built-in data structure: Tuple, List, and Dictionary are useful integrated data structures provided by the language.
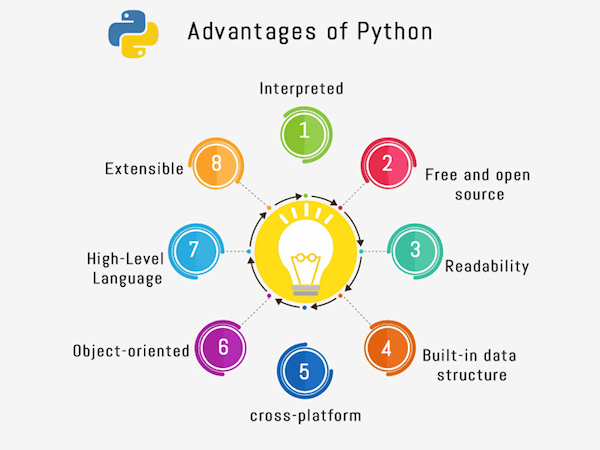
PEP 8 is defined as a document that helps us to provide the guidelines on how to write the Python code. It was written by Guido van Rossum, Barry Warsaw and Nick Coghlan in 2001.
It stands for Python Enhancement Proposal, and its major task is to improve the readability and consistency of Python code.
What do you mean by Python literals?
Literals can be defined as a data which is given in a variable or constant. Python supports the following literals:
String Literals
String literals are formed by enclosing text in the single or double quotes. For example, string literals are string values.
Example :
"Aman", '12345'.
Numeric Literals
Python supports three types of numeric literals integer, float and complex. See the examples.
Boolean Literals
Boolean literals are used to denote boolean values. It contains either True or False.
A function is a section of the program or a block of code that is written once and can be executed whenever required in the program. A function is a block of self-contained statements which has a valid name, parameters list, and body. Functions make programming more functional and modular to perform modular tasks. Python provides several built-in functions to complete tasks and also allows a user to create new functions as well.
There are two types of functions:
- Built-In Functions: copy(), len(), count() are the some built-in functions.
- User-defined Functions: Functions which are defined by a user known as user-defined functions.
Example: A general syntax of user defined function is given below.
Python zip() function returns a zip object, which maps a similar index of multiple containers. It takes an iterable, convert into iterator and aggregates the elements based on iterables passed. It returns an iterator of tuples.
Signature
Parameters
iterator1, iterator2, iterator3: These are iterator objects that are joined together.
Return
It returns an iterator from two or more iterators.
Note: If the given lists are of different lengths, zip stops generating tuples when the first list ends. It means two lists are having 3, and 5 lengths will create a 3-tuple.
There are two parameters passing mechanism in Python:
- Pass by references
- Pass by value
By default, all the parameters (arguments) are passed "by reference" to the functions. Thus, if you change the value of the parameter within a function, the change is reflected in the calling function as well. It indicates the original variable. For example, if a variable is declared as a = 10, and passed to a function where it?s value is modified to a = 20. Both the variables denote to the same value.
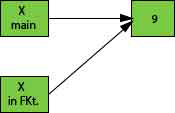
The pass by value is that whenever we pass the arguments to the function only values pass to the function, no reference passes to the function. It makes it immutable that means not changeable. Both variables hold the different values, and original value persists even after modifying in the function.
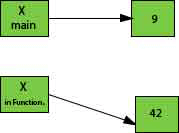
Python has a default argument concept which helps to call a method using an arbitrary number of arguments.
Python's constructor: _init__ () is the first method of a class. Whenever we try to instantiate an object __init__() is automatically invoked by python to initialize members of an object. We can't overload constructors or methods in Python. It shows an error if we try to overload.
You can use the remove() function to delete a specific object in the list.
If you want to delete an object at a specific location (index) in the list, you can either use del or pop.
Note: You don't need to import any extra module to use these functions for removing an element from the list.
We cannot use these methods with a tuple because the tuple is different from the list.