Interview :: C++
Friend function acts as a friend of the class. It can access the private and protected members of the class. The friend function is not a member of the class, but it must be listed in the class definition. The non-member function cannot access the private data of the class. Sometimes, it is necessary for the non-member function to access the data. The friend function is a non-member function and has the ability to access the private data of the class.
To make an outside function friendly to the class, we need to declare the function as a friend of the class as shown below:
Following are the characteristics of a friend function:
- The friend function is not in the scope of the class in which it has been declared.
- Since it is not in the scope of the class, so it cannot be called by using the object of the class. Therefore, friend function can be invoked like a normal function.
- A friend function cannot access the private members directly, it has to use an object name and dot operator with each member name.
- Friend function uses objects as arguments.
Let's understand this through an example:
Output:
11
- A virtual function is used to replace the implementation provided by the base class. The replacement is always called whenever the object in question is actually of the derived class, even if the object is accessed by a base pointer rather than a derived pointer.
- A virtual function is a member function which is present in the base class and redefined by the derived class.
- When we use the same function name in both base and derived class, the function in base class is declared with a keyword virtual.
- When the function is made virtual, then C++ determines at run-time which function is to be called based on the type of the object pointed by the base class pointer. Thus, by making the base class pointer to point different objects, we can execute different versions of the virtual functions.
Rules of a virtual function:
- The virtual functions should be a member of some class.
- The virtual function cannot be a static member.
- Virtual functions are called by using the object pointer.
- It can be a friend of another class.
- C++ does not contain virtual constructors but can have a virtual destructor.
You can answer this question in three manners:
- Never
- Rarely
- If you find that the problem domain cannot be accurately modeled any other way.
A Destructor is used to delete any extra resources allocated by the object. A destructor function is called automatically once the object goes out of the scope.
Rules of destructor:
- Destructors have the same name as class name and it is preceded by tilde.
- It does not contain any argument and no return type.
It is a type of arithmetical error. It happens when the result of an arithmetical operation been greater than the actual space provided by the system.
- When a single object behaves in many ways is known as overloading. A single object has the same name, but it provides different versions of the same function.
- C++ facilitates you to specify more than one definition for a function name or an operator in the same scope. It is called function overloading and operator overloading respectively.
- Overloading is of two types:
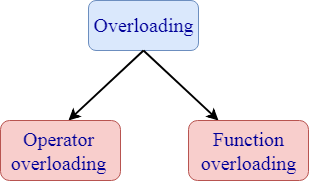
1. Operator overloading: Operator overloading is a compile-time polymorphism in which a standard operator is overloaded to provide a user-defined definition to it. For example, '+' operator is overloaded to perform the addition operation on data types such as int, float, etc.
Operator overloading can be implemented in the following functions:
- Member function
- Non-member function
- Friend function
Syntax of Operator overloading:
2. Function overloading: Function overloading is also a type of compile-time polymorphism which can define a family of functions with the same name. The function would perform different operations based on the argument list in the function call. The function to be invoked depends on the number of arguments and the type of the arguments in the argument list.
If you inherit a class into a derived class and provide a definition for one of the base class's function again inside the derived class, then this function is called overridden function, and this mechanism is known as function overriding.
Virtual inheritance facilitates you to create only one copy of each object even if the object appears more than one in the hierarchy.
A Constructor is a special method that initializes an object. Its name must be same as class name.
The "delete" operator is used to release the dynamic memory created by "new" operator.