Interview :: Node.js
npm stands for Node Package Manager. Following are the two main functionalities of npm:
- Online repositories for node.js packages/modules which are searchable on search.nodejs.org
- Command line utility to install packages, do version management and dependency management of Node.js packages.
Following is a list of tools that can be used in developing code in teams, to enforce a given style guide and to catch common errors using static analysis.
- JSLint
- JSHint
- ESLint
- JSCS
Operational errors are not bugs, but create problems with the system like request timeout or hardware failure. On the other hand, programmer errors are actual bugs.
What is the difference between the global installation of dependencies and local installation of dependencies?
- Global installation of dependencies is stored in /npm directory. While local installation of dependencies stores in the local mode. Here local mode refers to the package installation in node_modules directory lying in the folder where Node application is present.
- Globally deployed packages cannot be imported using require() in Node application directly. On the other hand, locally deployed packages are accessible via require().
- To install a Node project globally -g flag is used.
- To install a Node project locally, the syntax is:
The Node.js Assert is a way to write tests. It provides no feedback when running your test unless one fails. The assert module provides a simple set of assertion tests that can be used to test invariants. The module is intended for internal use by Node.js, but can be used in application code via require ('assert'). For example:
The Streams are the objects that facilitate you to read data from a source and write data to a destination. There are four types of streams in Node.js:
- Readable: This stream is used for reading operations.
- Writable: This stream is used for write operations.
- Duplex: This stream can be used for both reading and write operations.
- Transform: It is a type of duplex stream where the output computes according to input.
In Node.js, event-driven programming means as soon as Node starts its server, it initiates its variables, declares functions and then waits for an event to occur. It is one of the reasons why Node.js is pretty fast compared to other similar technologies.
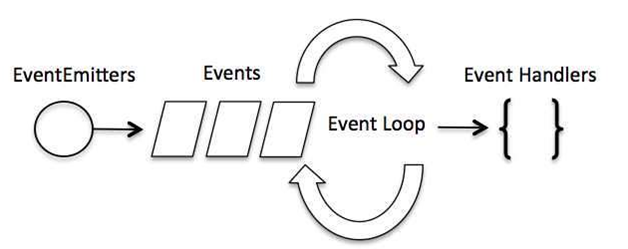
Although, Events and Callbacks look similar the differences lies in the fact that callback functions are called when an asynchronous function returns its result whereas event handling works on the observer pattern. Whenever an event gets fired, its listener function starts executing. Node.js has multiple in-built events available through the events module and EventEmitter class which is used to bind events and event listeners.
The Punycode is an encoding syntax which is used to convert Unicode (UTF-8) string of characters to ASCII string of characters. It is bundled with Node.js v0.6.2 and later versions. If you want to use it with other Node.js versions, then use npm to install Punycode module first. You have to used require ('Punycode') to access it.
Syntax:
The Node.js TTY module contains tty.ReadStream and tty.WriteStream classes. In most cases, there is no need to use this module directly. You have to used require ('tty') to access this module.