Interview :: Core Java
The object cloning is used to create the exact copy of an object. The clone() method of the Object class is used to clone an object. The java.lang.Cloneable interface must be implemented by the class whose object clone we want to create. If we don't implement Cloneable interface, clone() method generates CloneNotSupportedException.
Method overloading is the polymorphism technique which allows us to create multiple methods with the same name but different signature. We can achieve method overloading in two ways.
- By Changing the number of arguments
- By Changing the data type of arguments
Method overloading increases the readability of the program. Method overloading is performed to figure out the program quickly.
In Java, method overloading is not possible by changing the return type of the program due to avoid the ambiguity.
Test it NowOutput:
Compile Time Error: method add(int, int) is already defined in class Adder
No, We cannot overload the methods by just applying the static keyword to them(number of parameters and types are the same). Consider the following example.
Output
Animal.java:7: error: method consume(int) is already defined in class Animal static void consume(int a) ^ Animal.java:15: error: non-static method consume(int) cannot be referenced from a static context Animal.consume(20); ^ 2 errors
By Type promotion is method overloading, we mean that one data type can be promoted to another implicitly if no exact matching is found.
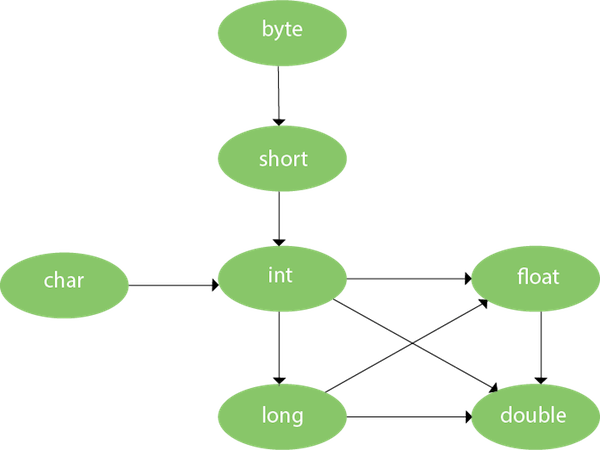
As displayed in the above diagram, the byte can be promoted to short, int, long, float or double. The short datatype can be promoted to int, long, float or double. The char datatype can be promoted to int, long, float or double and so on. Consider the following example.
Test it NowOutput
40 60
If a subclass provides a specific implementation of a method that is already provided by its parent class, it is known as Method Overriding. It is used for runtime polymorphism and to implement the interface methods.
Rules for Method overriding
- The method must have the same name as in the parent class.
- The method must have the same signature as in the parent class.
- Two classes must have an IS-A relationship between them.
No, you can't override the static method because they are the part of the class, not the object.
It is because the static method is the part of the class, and it is bound with class whereas instance method is bound with the object, and static gets memory in class area, and instance gets memory in a heap.
Yes.