Interview :: Angular JS
AngularJS controllers are used for:
- Setting the initial state of the $scope object
- Adding behavior to the $scope object
Data Binding is the automatic synchronization of data between model and view. In AngularJS, it performs the automatic synchronization process between the model and view.
If the model is changed, the view reflects it automatically and vice-versa. There are two ways of data binding that AngularJS supports:
- One Way Data Binding
In one-way data binding, view (UI part) does not get updated automatically when the data model is changed. We need to write custom codes to make it updated every time. A directive ng-bind has one-way data binding. Here, value is taken from the data model and inserted into an HTML element.
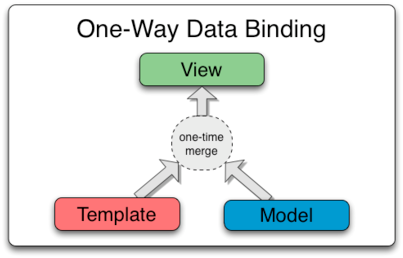
- Two Way Data Binding
In two-way data binding, scope variable changes its value whenever the data model is allotted to a different value. It treats the model as the single-source-of-truth in the application. The view is a projection of the model at all time s. If the model is changed, the view reflects the change and vice versa.
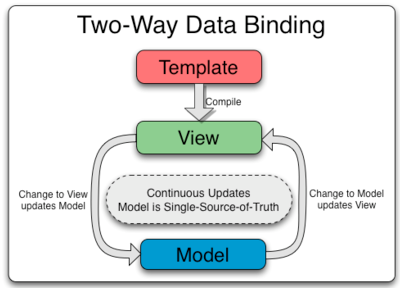
Services are objects that can be used to store and share data across the application. AngularJS offers many built-in services, and each of them is responsible for a specific task. They are always used with the prefix $ symbol.
Some of the important services used in any AngularJS application are as follows:
- $http- It is used to make an Ajax call to get the server data.
- $window- It provides a reference to a DOM object.
- $Location- It provides a reference to the browser location.
- $timeout- It provides a reference to the window.set timeout function.
- $Log- It is used for logging.
- $sanitize- It is used to avoid script injections and display raw HTML in the page.
- $Rootscope- It is used for scope hierarchy manipulation.
- $Route- It is used to display browser-based path in browser's URL.
- $Filter- It is used for providing filter access.
- $resource- It is used to work with Restful API.
- $document- It is used to access the window.Document object.
- $exceptionHandler- It is used for handling exceptions.
- $q- It provides a promise object.
- $cookies- It is used for reading, writing, and deleting the browser's cookies.
- $parse- It is used to convert an AngularJS expression into a function.
- $cacheFactory- It is used to evaluate the specified expression when the user changes the input.
A module is a container for the different parts of the application like a controller, services, filters, directives, etc. It is treated as a main() method. All the dependencies of applications are generally defined in modules only. A module is created using an angular object's module() method. For example:
Routing is one of the main features of the AngularJS framework, which is useful for creating a single page application (also referred to as SPA) with multiple views. It routes the application to different pages without reloading the application. In Angular, the ngRoute module is used to implement Routing. The ngView, $routeProvider, $route, and $routeParams are the different components of the ngRoute module, which help for configuring and mapping URL to views.
A template consists of HTML, CSS, and AngularJS directives, which are used to render the dynamic view. It is more like a static version of a web page with some additional properties to inject and render that data at runtime. The templates are combined with information coming from model and controller.
Expressions in AngularJS are the code snippets that resolve to a value. AngularJS expressions are placed inside {{expression}}. Expressions are included in the HTML elements.
AngularJS expressions can also contain various valid expressions similar to JavaScript expressions. We can also use the operators between numbers, including strings, literals, objects, and arrays inside the expression {{ }}.
For example:
AngularJS supports one-time binding expressions.
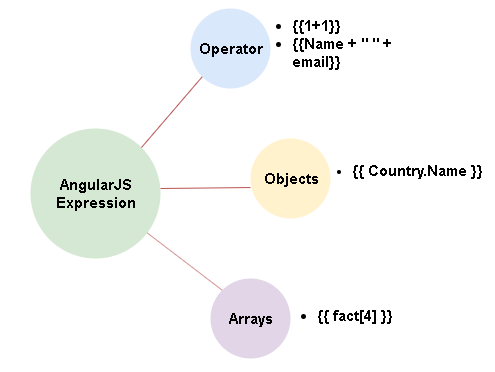
The key differences between the Angular expressions and JavaScript expressions are given below:
Angular Expressions | JavaScript Expressions |
---|---|
Angular expressions do not support conditional statements, loops, and exceptions. | JavaScript expressions support conditional statements, loops, and exceptions. |
Angular expressions support filters. | JavaScript expressions do not support filters. |
Angular expressions can be written inside HTML. | JavaScript expressions cannot be written inside HTML. |
What is the use of filter in AngularJS?
A filter is used to format the value of the expression to display the formatted output. AngularJS allows us to write our own filter. Filters can be added to expressions by using the pipe character |, followed by a filter. For example:
Filters can be applied in view templates, controllers, services and directives. It is important to know that filters are case-sensitive. There are some built-in filters provided by AngularJS such as Currency, Date, Filter, JSON, Limit, Lowercase, Number, Orderby, and Uppercase.
Uppercase filters are used to convert a text to upper case text. For example:
In above example, uppercase filter is added to an expression using pipe character. It will print student name in capital letters.
On the other side, lowercase filters are used to convert a text to lower case text. For example:
It will print student name in lowercase letters.